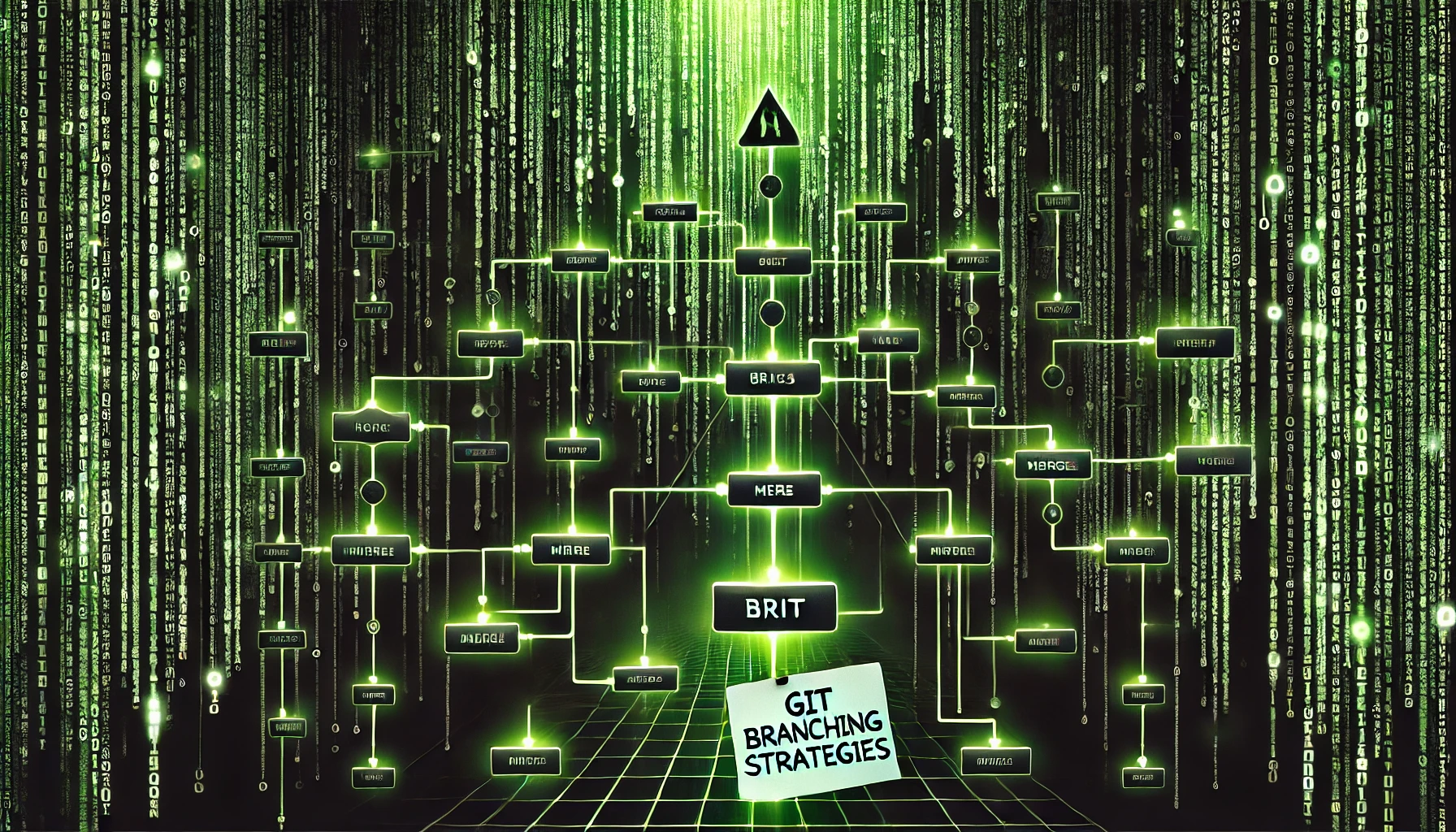
Git Branching Strategies
Best Practices for Git Branching Strategies and Release Management
Version control is a critical aspect of software development. It ensures that teams can work collaboratively without stepping on each other’s toes, manage releases effectively, and maintain high code quality. This article dives into best practices for Git branching strategies and release management to streamline development workflows.
Understanding Git Branching Strategies
A robust branching strategy ensures that teams can develop features, fix bugs, and prepare for releases simultaneously while maintaining a stable codebase. Below are commonly used branching models and best practices.
1. Git Flow
Git Flow is a time-tested strategy that introduces multiple branch types to organize work. It is best suited for projects with scheduled release cycles.
Branch Types:
main
: Always contains the production-ready code.develop
: The integration branch for features.feature/
: For developing individual features.release/
: Prepares a release with bug fixes and stabilization.hotfix/
: For critical fixes applied tomain
.
Flow Diagram:
Best Practices:
- Keep
main
always production-ready. - Frequently merge
develop
tofeature
branches to prevent divergence. - Use
release
branches to finalize work for deployment. - Limit
hotfix
branches to critical production issues.
2. GitHub Flow
GitHub Flow is a simpler alternative to Git Flow, designed for teams practicing continuous deployment.
Branch Types:
main
: Contains production-ready code.feature/
: For individual features or bug fixes. Merged intomain
after a pull request (PR).
Flow Diagram:
Best Practices:
- Deploy directly from
main
after PR reviews. - Use CI/CD pipelines to ensure
main
is always deployable. - Use descriptive branch names, e.g.,
feature/login-button
.
3. Trunk-Based Development
Trunk-Based Development focuses on a single branch (main
) where all changes are integrated. Developers work in short-lived branches or directly commit to the main
branch.
Branch Types:
main
: The single source of truth.- Short-lived branches: Created for individual tasks, often lasting a day or two.
Flow Diagram:
Best Practices:
- Commit frequently to avoid large, complex merges.
- Use feature flags to manage incomplete features.
- Rely on automated testing to maintain stability.
Release Management
Release management is about delivering high-quality software to users. The following practices integrate seamlessly with Git branching strategies.
1. Versioning
Adopt semantic versioning (MAJOR.MINOR.PATCH
):
- Increment MAJOR for incompatible changes.
- Increment MINOR for backward-compatible features.
- Increment PATCH for bug fixes.
2. Automate CI/CD Pipelines
Automation ensures that your main
branch is always deployable:
- Build: Compile and run tests automatically.
- Test: Use unit, integration, and end-to-end tests.
- Deploy: Push artifacts to staging and production environments.
3. Use Tags for Releases
Tagging helps you track and roll back to specific releases.
git tag -a v1.0.0 -m "Release version 1.0.0"
git push origin v1.0.0
4. Release Branch Freeze
During release preparation, freeze the branch to avoid unexpected changes:
- No new features, only bug fixes and stabilization.
- Merge fixes into both
release/
anddevelop
.
Choosing the Right Strategy
Project Type | Recommended Strategy |
---|---|
Large teams, scheduled releases | Git Flow |
Continuous deployment | GitHub Flow |
Rapid iteration, small teams | Trunk-Based |
Conclusion
The best branching strategy and release management practices depend on your team’s size, workflow, and release cadence. By following these principles, you can ensure streamlined development, maintain code quality, and deliver features to users efficiently.